As I am trying to learn Python, and probably will continue to do it for some time, I came across an issue that I think most of the network folks encountered and I thought to try and do it better and not manually. I am guessing everybody at some point had to close all the ports that are not used on a switch. The best practice for this case would be, shutdown the port and assign it to quarantine VLAN. This is more of a junior task, right? But when you have a lot of switches this kind of work takes a lot of time. Not to mention you might shutdown something else. As always, automation can help you. So I’ve chosen to do this with Python2.7(and pyeapi library ) on a couple of Arista switches.
The script was made to administratively shutdown ports (Ethernet, not Management) that are down and that are in access mode or trunk. But the script can be easily modified to shutdown ports that are in a particular VLAN or have some particular description for example. The port does not have to be in a down state(notconnect). You might choose to shutdown ports that are in vlan100 and are also ‘UP’ for example.
Below you have the variables I’ve defined. The state variable can be ‘connected’ if you want to shut down a port that it’s up.
# vars
switches = ['10.0.0.11','10.0.0.12']
""" the 3 types of ports: bridged,trunk,routed """
interface_mode = ['bridged','trunk']
""" notconnect is a port that is down """
state = 'notconnect'
Function to get the status of the ports:
def show_ports(switch):
node = pyeapi.connect(transport="https", host=switch, username="admin", password="admin", port=None)
interface_status = node.execute(["show interfaces status "])
return interface_status
Function to shutdown the ports and put them in a quarantine vlan999:
def shutdown_port(m,n):
try:
switch = Server("https://admin:admin@"+n+"/command-api")
response = switch.runCmds(1, ["enable", "configure", "interface " + m ,"shutdown","switchport access vlan 999" ,"switchport mode access"])
except:
print("Could not connect to {} to shutdown ports".format(n))
Main function:
def main():
for switch in switches:
try:
interface_status=show_ports(switch)
except:
print("Could not connect to {} to show interfaces".format(switch))
status = interface_status["result"][0]["interfaceStatuses"]
for n in status.keys():
a = status[n]["linkStatus"]
ifmode = interface_status["result"][0]["interfaceStatuses"][n]["vlanInformation"]["interfaceMode"]
if a == state and "Ethernet" in n and ifmode in interface_mode:
shutdown_port(n,switch)
As you see in the main function, I check every switch for the ports that have the state equal to variable ‘state’ and also are Ethernet ports and they are in a mode that is defined by the list ‘interface_mode’. In this case ‘trunk’ and ‘bridge’ (access mode). There is also ‘routed’ if you want to add it to the list. But you can choose other things to filter ports that you want to shutdown. For example the vlanId or the description.
Here you have all the possible things you can use to filter on:
{u'Ethernet1': {u'autoNegotiateActive': False,
u'autoNegotigateActive': False,
u'bandwidth': 0,
u'description': u'',
u'duplex': u'duplexFull',
u'interfaceType': u'EbraTestPhyPort',
u'lineProtocolStatus': u'up',
u'linkStatus': u'connected',
u'vlanInformation': {u'interfaceForwardingModel': u'bridged',
u'interfaceMode': u'bridged',
u'vlanId': 888}},
As an example, let’s say we want to shutdown all ports that have this description “ClientX”. We assume ClientX has left so we need to make sure all the ports he was using will be shutdown till we assign them to some other client. To do this I’ll create a variable to put the string i am interested in and I’ll also change the if conditional to filter based on the description:
#vars
description = 'ClientX'
def main():
...
if desc == description:
shutdown_port(n,switch)
The result looks like this (before/after):
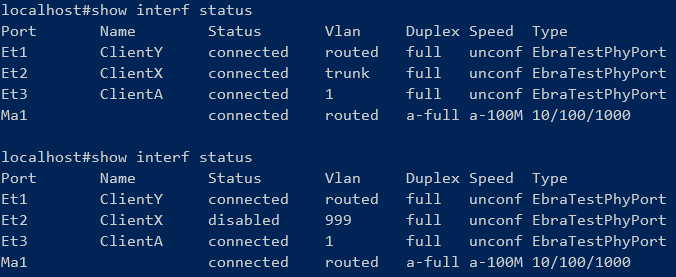
You can find the script here:
https://github.com/czirakim/Python.Arista.shutdown_ports